Remuxing a file¶
While remuxing, we take the encoded streams and mux
them into a different container. It is different from transcoding because transcoding involves re-encoding the streams, while remuxing involves just changing the containers without changing the encoding.
Encoding is a CPU-intensive task and as we don’t have to do that when we are remuxing, remuxing is a much faster operation than transcoding.
Graphically, this is how the re-muxing process looks like:
The “demuxing” part is handled by VLC internally and hence we don’t have to mention it in the code. Both the other two parts (mux
and access
) can be specified in the std
module.
One important thing to keep in mind is that because we are not “re-encoding” the streams, the original codecs should be compatible with the new muxer for the remuxing to work.
To know which codecs have been used to encode a media file, play it in VLC and navigate to
.Examples of Re-muxing¶
Example 1: MP4 to MKV¶
Suppose you have an MP4
file which has been encoded by h264
, mp4a
, and subt
codecs. As these codecs are compatible with the MKV
container, we can easily re-mux this file by the following code:
$ vlc sample.mp4 --sout="#std{access=file, mux=mkv, dst=sample.mkv}"
We can compare the codecs of the original MP4 file and the newly created MKV file.
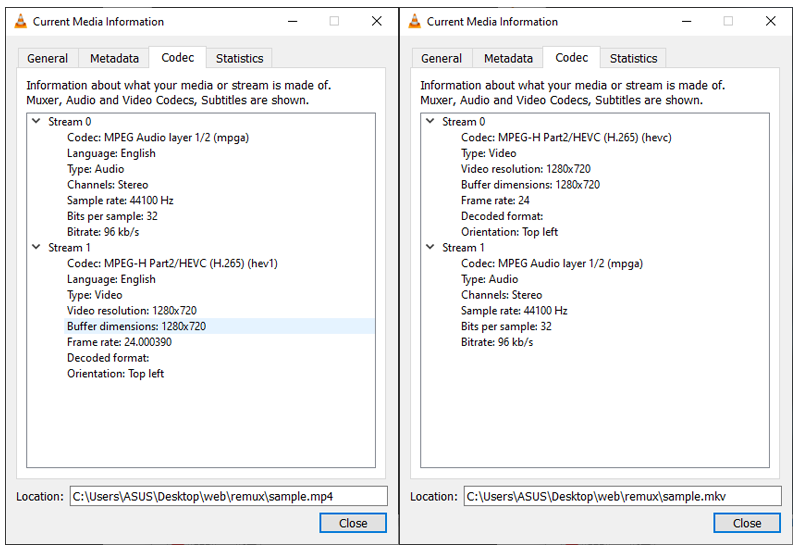
Notice that the same codecs have been used for encoding both the files.¶
To take a look into more details, we can use “MediaInfo”, an open source cross platform tool that displays technical information about media files. Following are the snippets of the general information for the same two files:
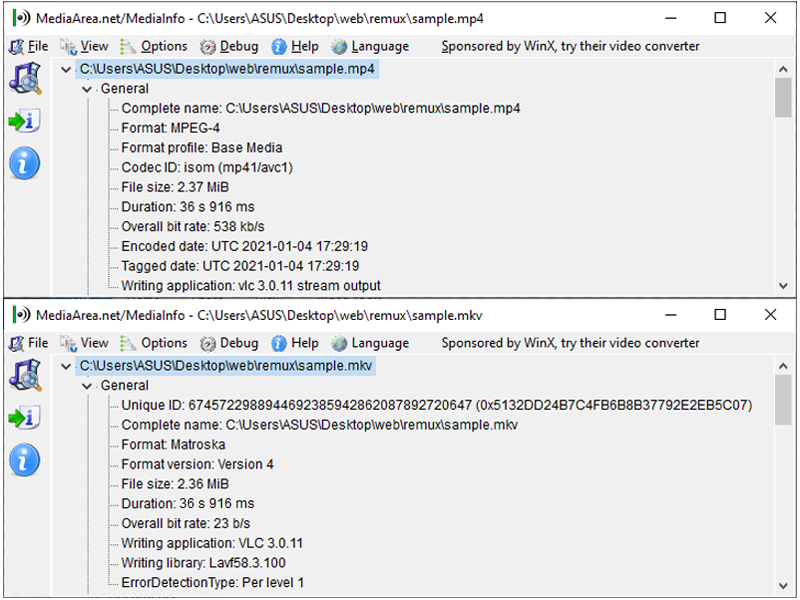
Notice that the container formats are different even though the same codecs are used.¶
This example is also useful in understanding the basic difference between transcoding and remuxing:
Transcoding involves re-encoding (with or without chainging the container), while
Remuxing involves just changing the container (without changing the encoding).
Further, the file size remains almost the same in remuxing as we don’t change the encoding. However, as it can be seen in Case 1 of trascoding, the file sizes can change substantially depending upon which codecs are used for re-encoding when transcoding a file.
Example 2: MKV to OGG¶
If you want to remux an MKV file (which had been encoded by theo
and vorb
codecs) into an OGG container, you can do that by running the following code:
$ vlc sample.mkv --sout="#std{access=file, mux=mkv, dst=sample.ogg}"
However, if the MKV file was encoded by theo
and mp4a
codecs, then you will not be able to re-mux it into an OGG container (as OGG is not compatible with the mp4a
codec). In this case, even if you run the above mentioned code it will not create any file.
This is why it is important to think about the codecs before re-muxing, because remuxing will only be successful if the original codecs and the new muxer (container) are compatible with each other.
Example 3: 3GP to MKV¶
Suppose you have a 3GP audio-only file which contains an AAC stream encoded by mp4a
codec. In this case, the codec-info of the file would look something like this:
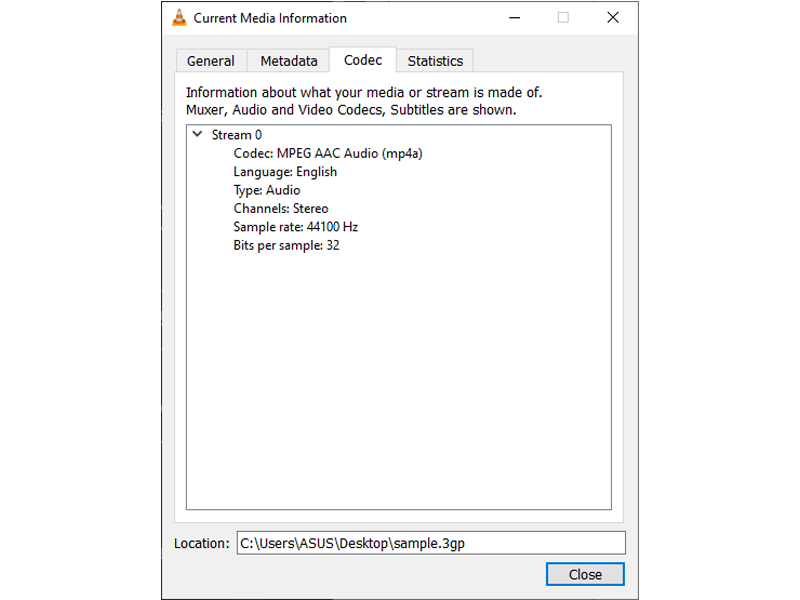
Following is a snippet of the compatability matrix for the Matroska container. It is not exhaustive and lists only a few of the popular codecs that are compatible with Matroska. You can pick any combination of acodec
, vcodec
, and scodec
from the table and mux them into the MKV container.
Audio codecs |
Video codecs |
Subtitle codecs |
---|---|---|
mpga |
h265 |
subt |
mp4a |
h264 |
ssa |
flac |
vp9 |
spu |
vorb |
mpgv |
|
a52 |
mp4v |
As we can see, AAC stream encoding (mp4a
acodec) is compatible with the MKV container. Hence, we can easily re-mux the file to an MKV container by running the following code:
$ vlc sample.3gp --sout="#std{access=file, mux=mkv, dst=sample.mkv}"